Lab 8.2
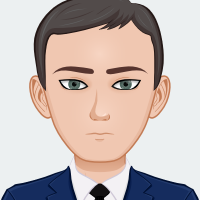
Is there a solution to this lab?
As far as I understand you cannot create a promise for an async function that has no callback. Without adapting the three functions you cannot complete this assignment (unless I am misreading the "Call the functions in such a way that A then B then C is printed out" and you are allowed to change them to a Promised version)?
If it can be done, a small hint (not the solution) would be appreciated
Comments
-
I can confirm you it can be done without modify the functions, but I'm so bad explaining it without giving you the solution.
I gonna try:
You have 3 functions opA, opB, opC. Transform they with the promisify util. Then do your things.
Hope this help, if you want my solution send me a message.1 -
Thanks xwarzdev. I finally managed. I had been playing around with promisying these functions, but didn't manage that when using a
.then
construct. Finally with using an additional async function in which I use three awaits, and then it works.Still puzzling why the
.then
construct is failing (i.e. it shows them in the parallel order of C, B, A)1 -
@mbaltus if you'd like to post the
.then
code I can take a look0 -
-
I got it to work with
then
- basically 'nesting' them - so it's kind of back to the downside of nested CBs/CB Hell 🤷🏽♂️0 -
I am only able to complete the lab without using promisify. Here is my code:
'use strict'
const { promisify } = require('util')const print = (err, contents) => {
if (err) console.error(err)
else console.log(contents)
}const opA = (cb) => {
return new Promise( (resolve, reject) =>
setTimeout(() => resolve(cb(null, 'A')), 500)
);
}const opB = (cb) => {
return new Promise( (resolve, reject) =>
setTimeout(() => resolve(cb(null, 'B')), 250)
);
}const opC = (cb) => {
return new Promise( (resolve, reject) =>
setTimeout(() => resolve(cb(null, 'C')), 125)
);
}async function run () {
await opA(print)
await opB(print)
await opC(print)
}run().catch(console.error)
I can't figure out how to do with promisify. Can anyone share the code?
1 -
is it allowed to modify the code already written in the file at the beginning of the exercise or should we use it as it is?
For instance, to promisify the opA, opB and opC functions, we need to modify them to be error-first callback style, like this:- const opA = (err, cb) => {
- setTimeout(() => {
- cb(null, "A");
- }, 500);
- };
For the purpose of the exercise, is that allowed or we should use the code as it is ?
0 -
HINT. Do not click Response if you do not want to check the response out
If it is allowed to modify the opX functions to make them error-first callback style functions, the following would be a valid response @davidmarkclements ?
Response>
```js "use strict"; const { promisify } = require("util"); const print = (err, contents) => { if (err) console.error(err); else console.log(contents); }; const opA = (err, cb) => { setTimeout(() => { cb(null, "A"); }, 500); }; const opB = (err, cb) => { setTimeout(() => { cb(null, "B"); }, 250); }; const opC = (err, cb) => { setTimeout(() => { cb(null, "C"); }, 125); }; const promiseOpA = promisify(opA); const promiseOpB = promisify(opB); const promiseOpC = promisify(opC); const run = async () => { const A = await promiseOpA(print); const B = await promiseOpB(print); const C = await promiseOpC(print); console.log(`${A}\n${B}\n${C}`); }; run(); ```0 -
@davidmarkclements said:
@mbaltus if you'd like to post the.then
code I can take a lookI managed this in the end. My error with the .then chaining, was that I tried to .then the print function as well. As this does not return a promiss, this is not allowed. By handling the print and the call to the next promissified op, I did manage to get it working.
So, to conclude, without changing opX and using promify, you can solve this one lab.
0 -
My code, for reference
Spoiler- 'use strict'
- const { promisify } = require('util')
- const print = (err, contents) => {
- if (err) console.error(err)
- else console.log(contents)
- }
- const opA = (cb) => {
- setTimeout(() => {
- cb(null, 'A')
- }, 500)
- }
- const opB = (cb) => {
- setTimeout(() => {
- cb(null, 'B')
- }, 250)
- }
- const opC = (cb) => {
- setTimeout(() => {
- cb(null, 'C')
- }, 125)
- }
- const printA = promisify(opA);
- const printB = promisify(opB);
- const printC = promisify(opC);
- printA().then((err, res) => {
- print(err,res)
- printB().then((err, res) => {
- print(err,res)
- printC().then((err, res) => {
- print(err,res)
- })
- })
- })
- // Alternative with an async caller function
- /*
- async function printer() {
- const resultA = await printA();
- print(null,resultA);
- const resultB = await printB();
- print(null,resultB);
- const resultC = await printC();
- print(null,resultC);
- }
- printer()
- */
- // Alternative with .then chaining
- //
0 -
good job everyone - that's correct. The function passed to promisify should have the signature (err, val) => {}.
0 -
@mbaltus and @davidmarkclements , my understanding is that when we use a function that takes a callback to create a new function that returns a promise. Instead of passing the original callback into the function we have to resolve the values of the returned promise. The values that will be resolved will be the values that were passed into the callback in the function definiton. Hence we will carryout this intention of passing those values to callback function calls.
(I am referring to the
print
function as a callback function even if it isn't being used as one in some instances.)I notice that @mbaltus when you used the
Promise.prototype.then()
you accidentally assigned the resolve parameter as the error paramter. As a result when you pass your resolved values into the callback function you passed the wrong values in.Although the error was hidden becuase the
print()
method conducts the same actions on each of its arguments it simply logs theerr
or thecontents
.@mbaltus I also noticed that you misused the
then()
method.then()
returns a promise. and so you donot need to write the code so it looks like 'callback hell' the idoiomatic way to write it is shown below in my code.@mbaltus I also noticed that you named the promisified functions print, I think that name is difficult to understand becuase the promisfied function return promsies therefore, I changed there names to
promiseA
promiseB
andpromiseC
.I also refactored some of the given code to my liking. I played around a lot.
.then solution
- 'use strict'
- const { promisify } = require('util')d
- const print = (err, contents) => {
- if (err) console.error(err+ ' this is an error');
- else console.log(contents + ' these are the contents');
- }
- const opA = (cb) => {
- setTimeout(() => cb(0, 'A'), 500)
- }
- const opB = (cb) => {
- setTimeout(() => cb(0, 'B'), 250)
- }
- const opC = (cb) => {
- setTimeout(() => cb(0, 'C'), 125)
- }
- const promiseA = promisify(opA);
- const promiseB = promisify(opB);
- const promiseC = promisify(opC);
- promiseA().then(res => {
- print(0, res);
- return promiseB();
- }).then(res => {
- print(0, res);
- return promiseC();
- }).then(res => {
- print(0, res);
- });
- /* OUTPUT
- A these are the contents
- B these are the contents
- C these are the contents
- */
async solution
- 'use strict'
- const { promisify } = require('util')
- const print = (err, contents) => {
- if (err) console.error(err+ ' this is an error');
- else console.log(contents + ' these are the contents');
- }
- const opA = (cb) => {
- setTimeout(() => cb(0, 'A'), 500)
- }
- const opB = (cb) => {
- setTimeout(() => cb(0, 'B'), 250)
- }
- const opC = (cb) => {
- setTimeout(() => cb(0, 'C'), 125)
- }
- let promiseA = promisify(opA);
- let promiseB = promisify(opB);
- let promiseC = promisify(opC);
- async function printer() {
- print(0, await promiseA());
- print(0, await promiseB());
- print(0, await promiseC());
- }
- printer();
- /* OUTPUT
- A these are the contents
- B these are the contents
- C these are the contents
- */
0 -
@andrewpartrickmiller thanks for pointing out that mistake. I struggled quite a bit with the .then construct and apparently fixed it incorrectly without noticing that (due to same output/result). I do see where the error is, and instead should add a .catch at the end to catch an err and pass that to print(err, null).
1 -
Great! This is fun, I really enjoy the interactions here.
0 -
Thank you everybody. I understand the way of both "promise" and "async"+"await".
0 -
good job everyone @andrewpartrickmiller is closest to correct and is correct for the exercise but don't forget error handling
when an awaited promise rejects in an async function it behaves like a throw (a promise rejection is an asynchronous exception).
To modify @andrewpartrickmiller code, wrap a try/catch around the awaits to do the error handling:
SPOILERS - reveal for code:
``` async function printer() { try { print(null, await promiseA()); print(null, await promiseB()); print(null, await promiseC()); } catch (err) { print(err); } } ```You can either wrap all of them in which case processing stops after the first failure or you can wrap each of them in a try/catch. For the exercise either way is fine. For real scenarios it depends on the situation.
4 -
One thing I'd like to know is how the callback is making its way into the async functions? We don't implicitly pass the callback "print" in anywhere yet results are obv getting returned to the print function and printed out
Spoiler- const a = promisify(opA);
- const b = promisify(opB);
- const c = promisify(opC);
- async function printSerial() {
- try {
- print(null, await a());
- print(null, await b());
- print(null, await c());
- } catch (e) {
- console.error(e);
- }
- }
If you console.log(cb) from within opA - we see that it is an anon func.
I think @andrewpartrickmiller touched on this a bit but I'm still wrapping my head around it. Maybe @davidmarkclements can help clarify
(Sorry if this is a double post - was having trouble with my first one.)
1 -
Here's the
util.promisify
implementation in Node core:If you remove the noise, the custom symbol stuff and property copying, it basically boils down to this:
- function promisify(original) {
- return function fn(...args) {
- return new Promise((resolve, reject) => {
- const callback = (err, value) => {
- if (err) {
- reject(err);
- } else {
- resolve(value);
- }
- }
- original(...args, callback);
- });
- }
- }
See the const there called
callback
- that's made by promisify and passed to the function you wrap (e.g. opA etc.).
So when the wrapped function calls it's callback function it calls that function inside the Promise executor function (https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise/Promise) which in turns calls the Promisesresolve
function.So if we we're to do this by hand with opA it would be:
- const opA = (cb) => { setTimeout(() => cb(null, 'A'), 500) }
- const a = () => new Promise((resolve, reject) => {
- opA((err, value) => {
- if (err) {
- reject(err)
- } else {
- resolve(err)
- }
- })
- })
Does that clarify it?
4 -
Yes! Thanks you @davidmarkclements!
1 -
@nayib said:
@davidmarkclementsis it allowed to modify the code already written in the file at the beginning of the exercise or should we use it as it is?
For instance, to promisify the opA, opB and opC functions, we need to modify them to be error-first callback style, like this:- const opA = (err, cb) => {
- setTimeout(() => {
- cb(null, "A");
- }, 500);
- };
For the purpose of the exercise, is that allowed or we should use the code as it is ?
Hi @nayib! that seems incorrect. I also got confused by the documentation at first but it's the callback itself that should be error-first not the function that is being promisified. In this case, the callback inside opA is already error first hence, no changes needed to the function.
Just mentioning this here just in case someone else gets confused as I did.
0 -
Hi everyone,
I thought that I could have an array of promises and iterate then in an async / await function but I cannot run it. it is missing something:
- 'use strict'
- const { promisify } = require('util')
- const print = (err, contents) => {
- if (err) console.error(err)
- else console.log(contents)
- }
- const opA = (cb) => {
- setTimeout(() => {
- cb(null, 'A')
- }, 500)
- }
- const opB = (cb) => {
- setTimeout(() => {
- cb(null, 'B')
- }, 250)
- }
- const opC = (cb) => {
- setTimeout(() => {
- cb(null, 'C')
- }, 125)
- }
- const ops = [
- promisify(opA),
- promisify(opB),
- promisify(opC)
- ]
- async function run(cb) {
- try {
- ops.forEach(op => cb(null, await op()))
- } catch (err) {
- console.error(err)
- }
- }
- run(print)
0 -
@jhortale the
ops.forEach
method accepts a function. You're trying toawait
in a function that isn't async.Use a for of loop instead and you'll be awaiting in the async
run
function instead0 -
Actually, the first thing I thought of for this lab, was to write my own helper which resolved to an array of values, instead of promisifying each function. Like so:
- const prom = (func) => {
- return new Promise((resolve, reject) => {
- func((err, val) => {
- if (err) {
- return reject(err);
- }
- return resolve(val);
- });
- });
- };
- Promise.all([prom(opA), prom(opB), prom(opC)]).then((res) => {
- res.forEach(print);
- });
0 -
@adbutterfield this is exactly what the
util.promisify
function does0 -
Thanks everyone! The exchange here gave me exactly what I needed to figure out where my misunderstanding was.
1 -
I used the below code. It worked for me but not sure how efficient it is.
- 'use strict'
- const { promisify } = require('util')
- const print = (err, contents) => {
- if (err) console.error(err)
- else console.log(contents)
- }
- const opA = (cb) => {
- setTimeout(() => {
- cb(null, 'A')
- }, 500)
- }
- const opB = (cb) => {
- setTimeout(() => {
- cb(null, 'B')
- }, 250)
- }
- const opC = (cb) => {
- setTimeout(() => {
- cb(null, 'C')
- }, 125)
- }
- const a = new Promise((resolve, reject) => {
- opA((err, contents) => {
- if (err) {
- reject(err)
- }
- else {
- print(err, contents)
- resolve(new Promise((resolveb, rejectb) => {
- opB((error,contents)=>{
- if(error)
- reject(error)
- else
- {
- print(error,contents)
- opC(print)
- }
- })
- }))
- }
- })
- }
- )
- a.then().catch((error)=>print(error,0))
0 -
@santhu3004 that defeats the purpose of using
util.promisify
, I'd also consider passing a promise to a resolve function, and not passing a function to a .then method as antipatterns. For the latter, if you're not passing a handler, why call .then, for the former, passing a promise to resolve instead of doing the work of the inner promise in the outer promise and then resolving the outer promise is .. more promises than you need.0 -
Agreed. Let me retry using promisfy
0 -
Hello,
Can someone please explain to me why this code still works and prints A, B, C?
- 'use strict';
- const { promisify } = require('util');
- const opA = (cb) => {
- setTimeout(() => {
- cb(null, 'A');
- }, 500);
- };
- const opB = (cb) => {
- setTimeout(() => {
- cb(null, 'B');
- }, 250);
- };
- const opC = (cb) => {
- setTimeout(() => {
- cb(null, 'C');
- }, 125);
- };
- const promA = promisify(opA);
- const promB = promisify(opB);
- const promC = promisify(opC);
- async function run() {
- try {
- console.log(await promA());
- console.log(await promB());
- console.log(await promC());
- } catch (err) {
- print(err);
- }
- }
- run();
Thank you
0 -
I think I got the answer myself. Basically what promisify function does it returns a Promise as has been said many times above.
- const a = () =>
- new Promise((resolve, reject) => {
- opA((err, value) => {
- if (err) {
- reject(err);
- } else {
- resolve(value);
- }
- });
- });
What I could not understand is that why it works without print function. It works because opA function:
- const opA = (cb) => {
- setTimeout(() => {
- cb(null, 'A');
- }, 500);
- };
takes a callback function cb which equals in our promise to this code:
- (err, value) => {
- if (err) {
- reject(err);
- } else {
- resolve(value);
- }
- }
and then in setTimeout functions it calls it with err equals to null and value equals to 'A'. Like that:
- const a = () =>
- new Promise((resolve, reject) => {
- opA((null, 'A') => {
- if (null) {
- reject(err);
- } else {
- resolve('A');
- }
- });
- });
so the promise resolves with the value of 'A'.
Hopefully it will help someone like me who is quite new to Javascript.
0
Categories
- All Categories
- 145 LFX Mentorship
- 145 LFX Mentorship: Linux Kernel
- 817 Linux Foundation IT Professional Programs
- 369 Cloud Engineer IT Professional Program
- 183 Advanced Cloud Engineer IT Professional Program
- 83 DevOps Engineer IT Professional Program
- 151 Cloud Native Developer IT Professional Program
- 143 Express Training Courses & Microlearning
- 143 Express Courses - Discussion Forum
- Microlearning - Discussion Forum
- 6.7K Training Courses
- 48 LFC110 Class Forum - Discontinued
- 73 LFC131 Class Forum
- 49 LFD102 Class Forum
- 238 LFD103 Class Forum
- 22 LFD110 Class Forum
- 45 LFD121 Class Forum
- 1 LFD123 Class Forum
- LFD125 Class Forum
- 18 LFD133 Class Forum
- 9 LFD134 Class Forum
- 18 LFD137 Class Forum
- 72 LFD201 Class Forum
- 5 LFD210 Class Forum
- 5 LFD210-CN Class Forum
- 2 LFD213 Class Forum - Discontinued
- 128 LFD232 Class Forum - Discontinued
- 2 LFD233 Class Forum
- 4 LFD237 Class Forum
- 24 LFD254 Class Forum
- 724 LFD259 Class Forum
- 111 LFD272 Class Forum - Discontinued
- 4 LFD272-JP クラス フォーラム
- 13 LFD273 Class Forum
- 259 LFS101 Class Forum
- 2 LFS111 Class Forum
- 3 LFS112 Class Forum
- 3 LFS116 Class Forum
- 7 LFS118 Class Forum
- 1 LFS120 Class Forum
- 9 LFS142 Class Forum
- 8 LFS144 Class Forum
- 4 LFS145 Class Forum
- 4 LFS146 Class Forum
- 16 LFS148 Class Forum
- 15 LFS151 Class Forum
- 5 LFS157 Class Forum
- 72 LFS158 Class Forum
- LFS158-JP クラス フォーラム
- 12 LFS162 Class Forum
- 2 LFS166 Class Forum
- 7 LFS167 Class Forum
- 3 LFS170 Class Forum
- 2 LFS171 Class Forum
- 3 LFS178 Class Forum
- 3 LFS180 Class Forum
- 2 LFS182 Class Forum
- 5 LFS183 Class Forum
- 34 LFS200 Class Forum
- 737 LFS201 Class Forum - Discontinued
- 3 LFS201-JP クラス フォーラム - Discontinued
- 21 LFS203 Class Forum
- 135 LFS207 Class Forum
- 2 LFS207-DE-Klassenforum
- 2 LFS207-JP クラス フォーラム
- 302 LFS211 Class Forum
- 56 LFS216 Class Forum
- 55 LFS241 Class Forum
- 50 LFS242 Class Forum
- 38 LFS243 Class Forum
- 16 LFS244 Class Forum
- 6 LFS245 Class Forum
- LFS246 Class Forum
- LFS248 Class Forum
- 113 LFS250 Class Forum
- 2 LFS250-JP クラス フォーラム
- 1 LFS251 Class Forum
- 157 LFS253 Class Forum
- 1 LFS254 Class Forum
- 2 LFS255 Class Forum
- 13 LFS256 Class Forum
- 1 LFS257 Class Forum
- 1.3K LFS258 Class Forum
- 11 LFS258-JP クラス フォーラム
- 135 LFS260 Class Forum
- 162 LFS261 Class Forum
- 43 LFS262 Class Forum
- 82 LFS263 Class Forum - Discontinued
- 15 LFS264 Class Forum - Discontinued
- 11 LFS266 Class Forum - Discontinued
- 24 LFS267 Class Forum
- 25 LFS268 Class Forum
- 37 LFS269 Class Forum
- 7 LFS270 Class Forum
- 202 LFS272 Class Forum - Discontinued
- 2 LFS272-JP クラス フォーラム
- 4 LFS147 Class Forum
- 2 LFS274 Class Forum
- 4 LFS281 Class Forum
- 18 LFW111 Class Forum
- 262 LFW211 Class Forum
- 186 LFW212 Class Forum
- 15 SKF100 Class Forum
- 1 SKF200 Class Forum
- 2 SKF201 Class Forum
- 797 Hardware
- 199 Drivers
- 68 I/O Devices
- 37 Monitors
- 104 Multimedia
- 174 Networking
- 91 Printers & Scanners
- 85 Storage
- 762 Linux Distributions
- 82 Debian
- 67 Fedora
- 18 Linux Mint
- 13 Mageia
- 23 openSUSE
- 149 Red Hat Enterprise
- 31 Slackware
- 13 SUSE Enterprise
- 355 Ubuntu
- 470 Linux System Administration
- 39 Cloud Computing
- 71 Command Line/Scripting
- Github systems admin projects
- 95 Linux Security
- 78 Network Management
- 102 System Management
- 47 Web Management
- 70 Mobile Computing
- 19 Android
- 38 Development
- 1.2K New to Linux
- 1K Getting Started with Linux
- 380 Off Topic
- 116 Introductions
- 177 Small Talk
- 26 Study Material
- 808 Programming and Development
- 304 Kernel Development
- 486 Software Development
- 1.8K Software
- 263 Applications
- 183 Command Line
- 3 Compiling/Installing
- 988 Games
- 317 Installation
- 104 All In Program
- 104 All In Forum
Upcoming Training
-
August 20, 2018
Kubernetes Administration (LFS458)
-
August 20, 2018
Linux System Administration (LFS301)
-
August 27, 2018
Open Source Virtualization (LFS462)
-
August 27, 2018
Linux Kernel Debugging and Security (LFD440)