Welcome to the Linux Foundation Forum!
fastify-reply-from with transform stream
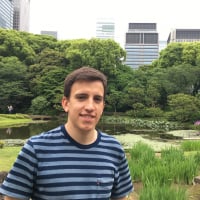
arturo.miguel.next
Posts: 7
Hello
Could someone explain to me why this does not work?
- 'use strict'
- const {Transform, Readable} = require('stream');
- const createTransformStream = () => {
- new Transform({
- transform(chunk, enc, next) {
- const uppercase = chunk.toString().toUpperCase();
- next(null, uppercase);
- }
- })
- }
- const uppercaseStream = createTransformStream();
- module.exports = async function (fastify, opts) {
- fastify.get('/', async function (request, reply) {
- const { url } = request.query;
- try {
- new URL(url)
- } catch (error) {
- throw fastify.httpErrors.badRequest()
- }
- await reply.from(url, {
- onResponse(req, reply, response) {
- reply.send(response.pipe(uppercaseStream));
- }
- });
- })
- }
Thanks
0
Comments
-
I forgot to return the Transform
- 'use strict'
- const {Transform, Readable} = require('stream');
- const createTransformStream = () => {
- return new Transform({
- transform(chunk, enc, next) {
- const uppercase = chunk.toString().toUpperCase();
- next(null, uppercase);
- }
- })
- }
- const uppercaseStream = createTransformStream();
- module.exports = async function (fastify, opts) {
- fastify.get('/', async function (request, reply) {
- const { url } = request.query;
- try {
- new URL(url)
- } catch (error) {
- throw fastify.httpErrors.badRequest()
- }
- await reply.from(url, {
- onResponse(req, reply, response) {
- reply.send(response.pipe(uppercaseStream));
- }
- });
- })
- }
0 -
nice catch @arturo.miguel.next
0 -
I wrote the code differently, but in all ways, the GET request from the client hangs forever.
However, sending the reply without any transformationreturn reply.from(url)
works as expected.the command
curl http://localhost:3000/?url=http://localhost:5050
from inside the server does not return any response either.any1 have an idea, why it's not working with me?
Thanks a lothere are two examples where the GET request is stuck forever:
- 'use strict'
- const { Transform, pipeline } = require('stream')
- function upper() {
- return new Transform({
- transform(chunk, encoding, callback) {
- callback(null, chunk.toString().toUpperCase())
- }
- })
- }
- module.exports = async function (fastify, opts) {
- fastify.get('/', async function (request, reply) {
- const { url } = request.query
- try {
- new URL(url)
- } catch (err) {
- throw fastify.httpErrors.badRequest()
- }
- console.log('Fetching from URL:', url)
- return reply.from(url, {
- onResponse (request, reply, res) {
- console.log('Received response from upstream URL')
- pipeline(res, upper(), reply.raw,
- (err) => {
- if (err) {
- console.error('Pipeline failed:', err)
- reply.code(500).send({ error: 'Internal Server Error' })
- } else {
- console.log('Pipeline succeeded')
- }
- }
- )
- }
- })
- })
- }
The original code as in the course also beating the same with me:
- 'use strict'
- const { Readable } = require('stream')
- async function * upper (res) {
- for await (const chunk of res) {
- yield chunk.toString().toUpperCase()
- }
- }
- module.exports = async function (fastify, opts) {
- fastify.get('/', async function (request, reply) {
- const { url } = request.query
- try {
- new URL(url)
- } catch (err) {
- throw fastify.httpErrors.badRequest()
- }
- return reply.from(url, {
- onResponse (request, reply, res) {
- reply.send(Readable.from(upper(res)))
- }
- })
- })
- }
0 -
for the bellow code, I get an error message "res is not async iterable"
- 'use strict'
- const { Readable } = require('stream')
- async function * upper (res) {
- for await (const chunk of res) {
- yield chunk.toString().toUpperCase()
- }
- }
- module.exports = async function (fastify, opts) {
- fastify.get('/', async function (request, reply) {
- const { url } = request.query
- try {
- new URL(url)
- } catch (err) {
- throw fastify.httpErrors.badRequest()
- }
- return reply.from(url, {
- onResponse (request, reply, res) {
- reply.send(Readable.from(upper(res)))
- }
- })
- })
- }
0
Categories
- All Categories
- 232 LFX Mentorship
- 232 LFX Mentorship: Linux Kernel
- 812 Linux Foundation IT Professional Programs
- 365 Cloud Engineer IT Professional Program
- 183 Advanced Cloud Engineer IT Professional Program
- 82 DevOps Engineer IT Professional Program
- 151 Cloud Native Developer IT Professional Program
- 140 Express Training Courses & Microlearning
- 140 Express Courses - Discussion Forum
- Microlearning - Discussion Forum
- 6.4K Training Courses
- 48 LFC110 Class Forum - Discontinued
- 71 LFC131 Class Forum
- 47 LFD102 Class Forum
- 229 LFD103 Class Forum
- 20 LFD110 Class Forum
- 44 LFD121 Class Forum
- LFD125 Class Forum
- 18 LFD133 Class Forum
- 8 LFD134 Class Forum
- 18 LFD137 Class Forum
- 71 LFD201 Class Forum
- 5 LFD210 Class Forum
- 5 LFD210-CN Class Forum
- 2 LFD213 Class Forum - Discontinued
- 128 LFD232 Class Forum - Discontinued
- 2 LFD233 Class Forum
- 4 LFD237 Class Forum
- 24 LFD254 Class Forum
- 712 LFD259 Class Forum
- 111 LFD272 Class Forum - Discontinued
- 4 LFD272-JP クラス フォーラム
- 13 LFD273 Class Forum
- 201 LFS101 Class Forum
- 1 LFS111 Class Forum
- 3 LFS112 Class Forum
- 3 LFS116 Class Forum
- 7 LFS118 Class Forum
- LFS120 Class Forum
- 9 LFS142 Class Forum
- 8 LFS144 Class Forum
- 4 LFS145 Class Forum
- 3 LFS146 Class Forum
- 15 LFS148 Class Forum
- 15 LFS151 Class Forum
- 5 LFS157 Class Forum
- 49 LFS158 Class Forum
- LFS158-JP クラス フォーラム
- 10 LFS162 Class Forum
- 2 LFS166 Class Forum
- 5 LFS167 Class Forum
- 3 LFS170 Class Forum
- 2 LFS171 Class Forum
- 3 LFS178 Class Forum
- 3 LFS180 Class Forum
- 2 LFS182 Class Forum
- 5 LFS183 Class Forum
- 33 LFS200 Class Forum
- 737 LFS201 Class Forum - Discontinued
- 3 LFS201-JP クラス フォーラム - Discontinued
- 19 LFS203 Class Forum
- 135 LFS207 Class Forum
- 2 LFS207-DE-Klassenforum
- 2 LFS207-JP クラス フォーラム
- 302 LFS211 Class Forum
- 56 LFS216 Class Forum
- 52 LFS241 Class Forum
- 50 LFS242 Class Forum
- 38 LFS243 Class Forum
- 16 LFS244 Class Forum
- 5 LFS245 Class Forum
- LFS246 Class Forum
- LFS248 Class Forum
- 54 LFS250 Class Forum
- 2 LFS250-JP クラス フォーラム
- 1 LFS251 Class Forum
- 156 LFS253 Class Forum
- 1 LFS254 Class Forum
- 1 LFS255 Class Forum
- 10 LFS256 Class Forum
- 1 LFS257 Class Forum
- 1.3K LFS258 Class Forum
- 11 LFS258-JP クラス フォーラム
- 135 LFS260 Class Forum
- 160 LFS261 Class Forum
- 43 LFS262 Class Forum
- 82 LFS263 Class Forum - Discontinued
- 15 LFS264 Class Forum - Discontinued
- 11 LFS266 Class Forum - Discontinued
- 24 LFS267 Class Forum
- 25 LFS268 Class Forum
- 32 LFS269 Class Forum
- 6 LFS270 Class Forum
- 202 LFS272 Class Forum - Discontinued
- 2 LFS272-JP クラス フォーラム
- 4 LFS147 Class Forum
- 1 LFS274 Class Forum
- 4 LFS281 Class Forum
- 15 LFW111 Class Forum
- 262 LFW211 Class Forum
- 184 LFW212 Class Forum
- 15 SKF100 Class Forum
- 1 SKF200 Class Forum
- 2 SKF201 Class Forum
- 797 Hardware
- 199 Drivers
- 68 I/O Devices
- 37 Monitors
- 104 Multimedia
- 174 Networking
- 91 Printers & Scanners
- 85 Storage
- 759 Linux Distributions
- 82 Debian
- 67 Fedora
- 17 Linux Mint
- 13 Mageia
- 23 openSUSE
- 148 Red Hat Enterprise
- 31 Slackware
- 13 SUSE Enterprise
- 354 Ubuntu
- 470 Linux System Administration
- 39 Cloud Computing
- 71 Command Line/Scripting
- Github systems admin projects
- 95 Linux Security
- 78 Network Management
- 102 System Management
- 47 Web Management
- 69 Mobile Computing
- 18 Android
- 38 Development
- 1.2K New to Linux
- 1K Getting Started with Linux
- 377 Off Topic
- 115 Introductions
- 175 Small Talk
- 26 Study Material
- 807 Programming and Development
- 304 Kernel Development
- 485 Software Development
- 1.8K Software
- 263 Applications
- 183 Command Line
- 3 Compiling/Installing
- 988 Games
- 317 Installation
- 103 All In Program
- 103 All In Forum
Upcoming Training
-
August 20, 2018
Kubernetes Administration (LFS458)
-
August 20, 2018
Linux System Administration (LFS301)
-
August 27, 2018
Open Source Virtualization (LFS462)
-
August 27, 2018
Linux Kernel Debugging and Security (LFD440)